In this second episode of the Making blockchain real Series, we take a look at Swapable: automated liquidity pools, and put light on some of the ideas that flourished from the Uniswap project that we have implemented in Swapable.
In case you don’t know us, Making blockchain real presents a Series of episodes about blockchain projects and reviews their programmability by explaining implementation details through simplistic showcases.
Table of contents
- What is an automated liquidity pool?
- Swapable: Automated liquidity pools
- How to create a liquidity pool with Swapable?
- How to swap currencies with Swapable?
- What are the next steps with Swapable?
- Benefit from blockchain for digital markets
What is an automated liquidity pool?
In essence, liquidity pools are pools of digital assets that are locked in a smart [digital] contract. These types of pools are used to facilitate trading by providing liquidity.
The main reason behind the introduction of liquidity pools, is that the order book exchange model relies heavily on having one – or multiple – market makers that are willing to always “make the market”, a.k.a. to provide liquidity for a digital asset.
With liquidity pools, market making is automated. Typically, liquidity pools hold funds in 2 distinct digital assets which, together, can be referred to as the market pair. When a new pool is created, the first liquidity provider decides on the market price for said digital assets that are in the pool.
When liquidity is supplied into a pool, the liquidity provider receives an amount of shares that is proportional to its contribution to the pool. This amount of shares can then be burned when the liquidity provider wants to cash out of its position.
Traders use liquidity pools to swap digital assets that are in market pairs. These swaps use a price adjustment according to a deterministic pricing algorithm, a.k.a. the constant product formula. The main takeaway here is that the ratio of digital assets in a liquidity pool dictates the price of the digital assets.
Swapable: Automated liquidity pools
The team at Using Blockchain Ltd has been hard at work on the authoring and implementation of an open standard for automated liquidity pools that make use of Symbol from NEM. It is with immense pleasure that we published the swapable v1.0.0 library over at NPM.
The swapable library is a first-of-a-kind implementation that is based on our previous token standards work from NIP13. The library implementation defines digital contracts that are compatible with any Symbol network to enable automated digital markets.
How to create a liquidity pool with Swapable?
Our implementation of liquidity pools differs from Uniswap – or similar automated market makers – in that the liquidity pools are managed through a BIP39 mnemonic seed. The deterministic account that is used to create liquidity pools should later be secured with multi-signature on-chain in order to enable DAO-like governance.
It is important to note that the implementation in Swapable presents a first light version of such self-sovereign automated liquidity pools. In other words, we have more following up for you, but we need to keep this article simple enough. (#soon)
How does this map to source code and Symbol from NEM, you ask? Following snippet of source code can be used to create a liquidity pool with Swapable:
/**
* This file is part of Swapable shared under AGPL-3.0
* Copyright (C) 2021 Using Blockchain Ltd, Reg No.: 12658136, United Kingdom
*/
import {MnemonicPassPhrase} from ‘symbol-hd-wallets’
import {TransactionUri} from ‘symbol-uri-scheme’
import {Swapable, Symbol, TransactionParameters} from ‘swapable’
// (1) initialize BIP39 mnemonic seed
const assetKeys = MnemonicPassPhrase.createRandom()
// (2) initialize Swapable library
const reader = new Symbol.Reader(…)
const signer = new Symbol.Signer()
const swapable = new Swapable.AutomatedPool(
‘SWP:XYM’,
reader,
signer,
assetKeys,
)
// (3) offline creation of the `CreatePool` digital contract
const poolId = swapable.create(
swapable.target.publicAccount, // actor
new PublicAccount(…), // liquidity provider
new AssetAmount(Symbol_Testnet_SWP, 10),
new AssetAmount(Symbol_Testnet_XYM, 10),
new TransactionParameters(…),
)
// (4) get the transaction URI for `CreatePool` execution
const resultURI: TransactionURI = swapable.result
Source: UsingBlockchain/Swapable open source library
Following along, let’s analyze the above snippet of Typescript source code. In (1), we create a random mnemonic pass phrase – note that this information must be safely backed up. Once we did that, we can initialize the swapable library as shown in (2).
The swapable library requires a pair of blockchain network adapters – i.e. those are used to interact with a blockchain network, in our example using Symbol from NEM. More blockchain adapters are planned to be added in the future. Back to our …horsesswaps.
In (3) comes the magic that creates a digital contract that can later be signed and executed using a Symbol from NEM blockchain network of your choice. You can see here that we have to define a liquidity provider which is the account that creates the liquidity pool. At execution, Swapable will distribute a first batch of automated pool shares to this liquidity provider.
The last step in the above snippet is optional, it permits to read the transaction URI that is being generated by Swapable. You can use any Symbol from NEM Wallet implementation to sign/announce the transaction, to create a liquidity pool on a connected blockchain network.
Note that the creation of digital contracts, as well as the digital signature creation, in relation with automated liquidity pools are always done offline.
How to swap currencies with Swapable?
After creating a first liquidity pool, it is time for the traders to play their game of …trading. Sounds simple, right? Well, it is. In fact, swapping currencies is defined as the action of swapping one digital asset for the other digital asset in a liquidity pool, adding a provider fee to the swapped amount.
The added liquidity provider fee is automatically distributed to liquidity providers, in the form of automated pool shares that can later be redeemed for [parts of] the liquidity that was added in a liquidity pool. Additionally, the provider fee ensures that the product of the amounts of digital asset present in a liquidity pool (i.e. the constant product), actually always increases when swaps are executed.
Thus, liquidity providers add liquidity into pools, and traders swap the digital assets between each other which, in turn, augments the constant product by adding a liquidity provider fee, thereby augmenting the liquidity of said market pair or liquidity pool (i.e. “market making”).
Let’s see how this maps to a snippet of source code, re-using the above declared swapable automated pool, we will now execute a swap of digital assets taking the position of a trader:
/**
* This file is part of Swapable shared under AGPL-3.0
* Copyright (C) 2021 Using Blockchain Ltd, Reg No.: 12658136, United Kingdom
*/
import {MnemonicPassPhrase} from ‘symbol-hd-wallets’
import {TransactionUri} from ‘symbol-uri-scheme’
import {Swapable, Symbol, TransactionParameters} from ‘swapable’
// (1) offline creation of the `Swap` digital contract
const resultURI: TransactionURI = swapable.execute(
swapable.target,
swapable.identifier,
‘Swap’,
new TransactionParameters(…),
[
new CommandOption(‘trader’, new PublicAccount(…)),
new CommandOption(‘input_x’, new AssetAmount(…)), // (2) defines an input asset amount and identifier
new CommandOption(‘output’, new AssetIdentifier(…)), // (3) defines an output asset identifier
],
)
// (4) get the transaction URI for `Swap` execution
const resultURI: TransactionURI = swapable.result
Source: UsingBlockchain/Swapable open source library
Nice and clean – let’s analyse the above snippet of Typescript source code. In (1), we re-use the first snippet’s swapable automated liquidity pool to execute a Swap digital contract.
Moreover, in (2) we define an input asset amount and identifier, this is the input of the swap – i.e. an amount of one of both digital assets in the liquidity pool. In (3), we define an asset identifier, this time we need only the identifier because the automated liquidity pool automatically determines the output asset amount.
The last step in the above snippet is optional, it permits to read the transaction URI that is being generated by Swapable. You can use any Symbol from NEM Wallet implementation to sign/announce the transaction, to create a liquidity pool on a connected blockchain network.
What are the next steps with Swapable?
This first version of swapable enables digital markets for Symbol from NEM. Given that we find enough support/sponsors, we aim to continue its’ development in the realm of open source because we believe that an open access to technological innovation is most important in a digitizing economy.
The next steps for swapable will be to integrate the library into one of our pilot projects – but psshhhh, this is still in the oven. In the mean time, you can use this open source library as you see fits! Please note that the author of the package cannot be held liable for any malintentioned usage or consequences of using this library.
Our team is striving for technological innovation and executes using innovative development skills, always open source. We are actively looking for sponsors, feel free to reach out to us on our Github Sponsors Profile.
Benefit from blockchain for digital markets
Blockchain can be used to define a base layer for digital markets, i.e. to improve security in markets and transparency around trading, or to enable easier digital access to digital markets. We think using blockchain for digital markets has the potential to enable great technological features. (Read more on how business can benefit from using blockchain)
You can use blockchain projects as an instrument of truth for digital markets. One, that is distributed and open for anyone to use. The incentivization strategies around blockchain networks may differ from one project to the other, but the principles of immutability of data and that of preserving data integrity are usually respected by all blockchain projects.
The full source code for the swapable library can be found in the repository: UsingBlockchain/Swapable at Github.
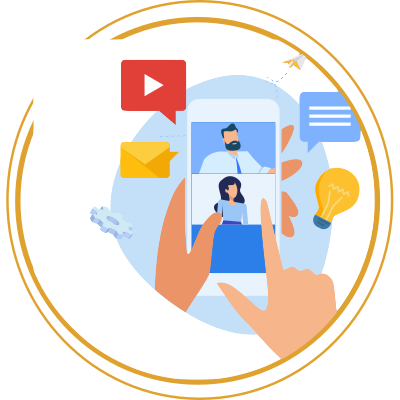
We hope that this article was insightful for you and are looking forward to any feedback and messages. Please share your thoughts in the comments section below!
Disclaimer
This website may contain information about financial firms, employees of such firms, and/or their products and services such as real estate, stocks, bonds, and other types of investments. While this website may intend - as the author deem necessary - to provide information on financial matters and investments, such information or references should not be construed or interpreted as investment advice or viewed as an endorsement.